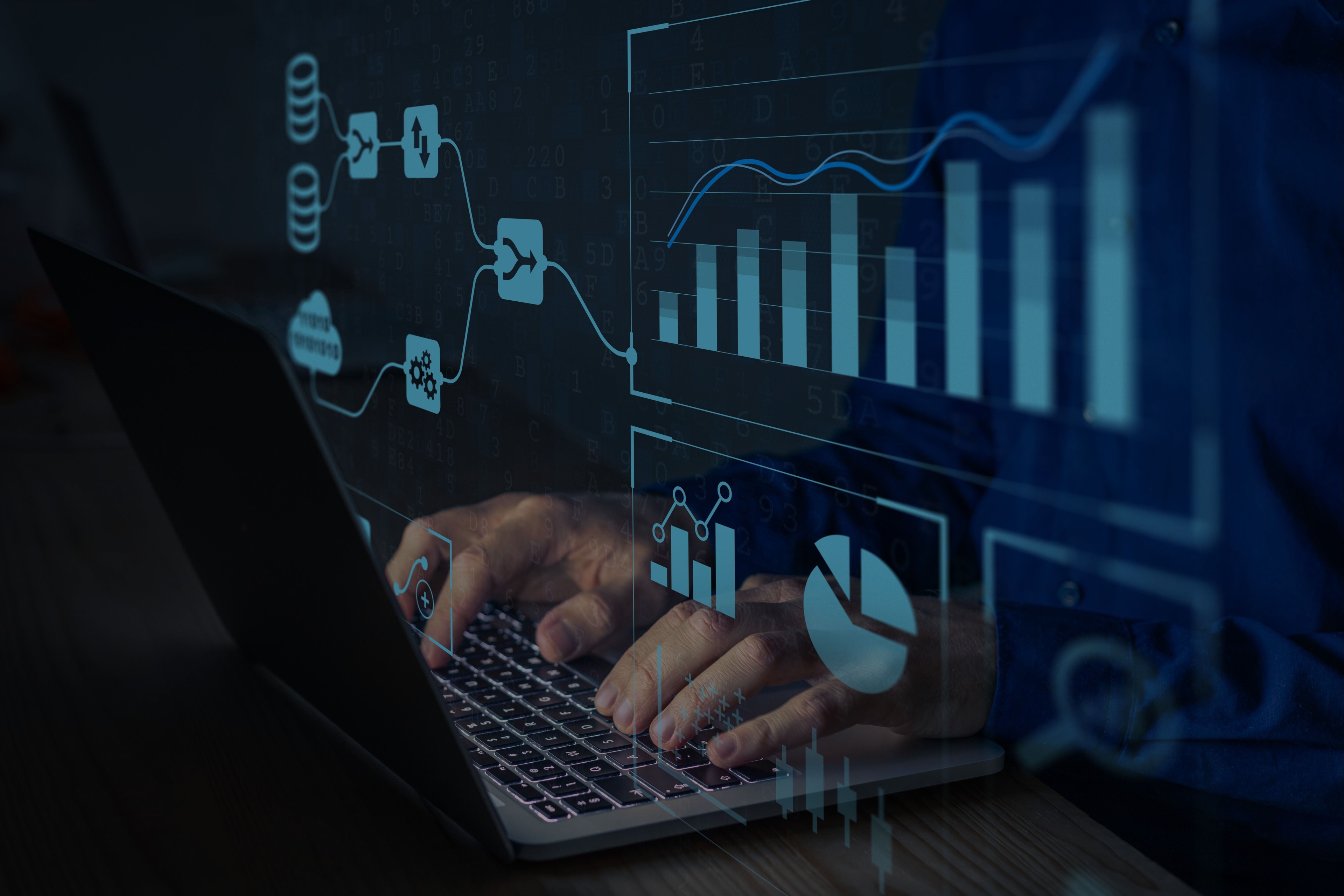
Unity での C# スクリプトのフォーマットのベストプラクティス
C# コードをフォーマットする正しい方法は 1 つだけではないかもしれませんが、チーム全体で一貫したスタイルに同意することで、よりクリーンで読みやすく、スケーラブルなコードベースを実現できます。このページでは、独自のスタイル ガイドを作成するときにクラス、メソッド、コメントに関して留意すべきヒントと重要な考慮事項について説明します。
注:ここで共有される推奨事項は、Microsoft が提供する推奨事項に基づいています。最適なコード スタイル ガイド ルールは、チームのニーズに合ったものです。
コードスタイルガイドの例は ここで 見つけられます。または、完全な電子書籍をダウンロードしてください。 C# スタイル ガイドを作成します。スケーラブルなクリーンなコードを書く。
コードのフォーマット
名前を付けるだけでなく、フォーマットも推測を減らし、コードの明確さを向上させるのに役立ちます。標準化されたスタイル ガイドに従うことで、コード レビューはコードの見た目ではなく、コードの動作に重点を置くようになります。
チームがコードをフォーマットする方法をカスタマイズすることを目指します。Unity スタイル ガイドを設定するときは、次の各コード フォーマットの提案を考慮してください。チームのニーズに合わせて、これらのサンプル ルールを省略、拡張、または変更することができます。
いずれの場合も、チームが各書式設定ルールをどのように実装するかを検討し、全員がそれを均一に適用するようにします。矛盾を解決するには、チームのスタイル ガイドを参照してください。フォーマットについてあまり考えなければ、より生産的かつ創造的に作業できるようになります。
書式設定のガイドラインをいくつか見てみましょう。
プロパティ
プロパティは、クラス値の読み取り、書き込み、または計算を行う柔軟なメカニズムを提供します。プロパティはパブリック メンバー変数のように動作しますが、実際には アクセサーと呼ばれる特別なメソッドです。
各プロパティには、 バッキング フィールドと呼ばれるプライベート フィールドにアクセスするための get メソッドと set メソッドがあります。この方法では、プロパティは データをカプセル化し、ユーザーまたは外部オブジェクトによる不要な変更からデータを隠します。「ゲッター」と「セッター」にはそれぞれ独自のアクセス修飾子があり、プロパティ を読み取り/書き込み、 読み取り専用、または 書き込み専用にすることができます。
アクセサーを使用して、データを検証または変換することもできます (たとえば、データが優先形式に適合していることを確認したり、値を特定の単位に変更したりするなど)。
プロパティの構文は異なる場合があるため、スタイル ガイドでその書式を定義する必要があります。コード内でプロパティの一貫性を保つためのヒントについては、次の例を参照してください。
式本体のプロパティ
単一行の読み取り専用プロパティには、 式本体のプロパティを使用します (=>):これはプライベート バッキング フィールドを返します。
自動実装プロパティ
その他はすべて 、式本体の { get; set; } 構文を使用します。バッキング フィールドを指定せずにパブリック プロパティを公開するだけの場合は、 自動実装プロパティを使用します。
set アクセサーと get アクセサーに式本体の構文を適用します。書き込みアクセスを提供したくない場合は、「setter」をプライベートにすることを忘れないでください。複数行のコード ブロックの場合は、閉じ括弧を開き括弧に揃えます。
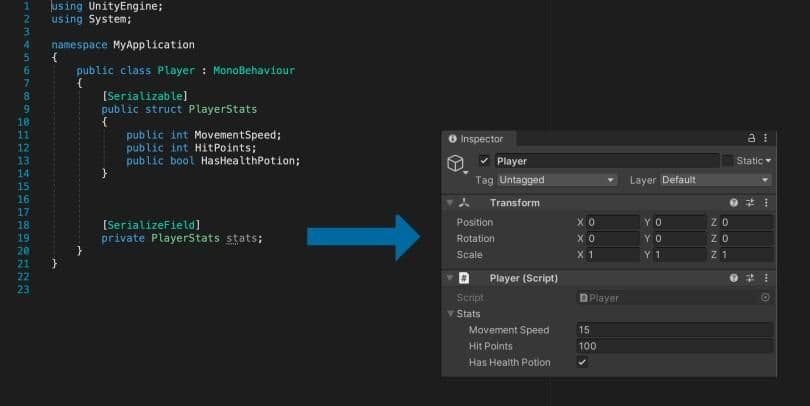
シリアル化
スクリプトのシリアル化は、データ構造またはオブジェクトの状態を、Unity が後で保存および再構築できる形式に変換する自動プロセスです。パフォーマンス上の理由から、Unity は他のプログラミング環境とは異なる方法でシリアル化を処理します。
シリアル化されたフィールドは インスペクターに表示されますが、静的、定数、または読み取り専用フィールドをシリアル化することはできません。これらはパブリックであるか、[SerializeField] 属性でタグ付けされている必要があります。Unity は特定のフィールド タイプのみをシリアル化するため、シリアル化ルールの完全なセットについては ドキュメント を参照してください。
シリアル化されたフィールドを操作するときは、次の基本的なガイドラインに従ってください。
[SerializeField]属性を使用します: SerializeField属性は、プライベート変数または保護された変数を操作して、それらをインスペクターに表示することができます。これにより、変数を public としてマークするよりも適切にデータがカプセル化され、外部オブジェクトがその値を上書きするのを防ぐことができます。
範囲属性を使用して最小値と最大値を設定します。[Range(min, max)]属性は、ユーザーが数値フィールドに割り当てることができる内容を制限する場合に便利です。また、フィールドをインスペクタのスライダーとして便利に表示することもできます。
シリアル化可能なクラスまたは構造体にデータをグループ化して、インスペクターをクリーンアップします。 パブリック クラスまたは構造体を定義し、[Serializable]属性でマークします。インスペクターで公開する各タイプのパブリック変数を定義します。
このシリアル化可能なクラスを別のクラスから参照します。結果の変数は、インスペクターの折りたたみ可能な単位内に表示されます。
括弧またはインデントのスタイル
C# には 2 つの一般的なインデント スタイルがあります。
Allman スタイルは、BSD スタイル (BSD Unix に由来) とも呼ばれ、開き中括弧を新しい行に配置します。
K&R スタイル、つまり「1 つの真の中括弧スタイル」では、開き中括弧が前のヘッダーと同じ行に配置されます。
これらのインデント スタイル にもバリエーションがあります。このガイドの例では 、Microsoft フレームワーク設計ガイドラインの Allman スタイルを使用しています。チームとしてどちらを選択するかに関係なく、全員が同じインデントと括弧のスタイルに従うようにしてください。次のセクションのヒントも試してみてください。
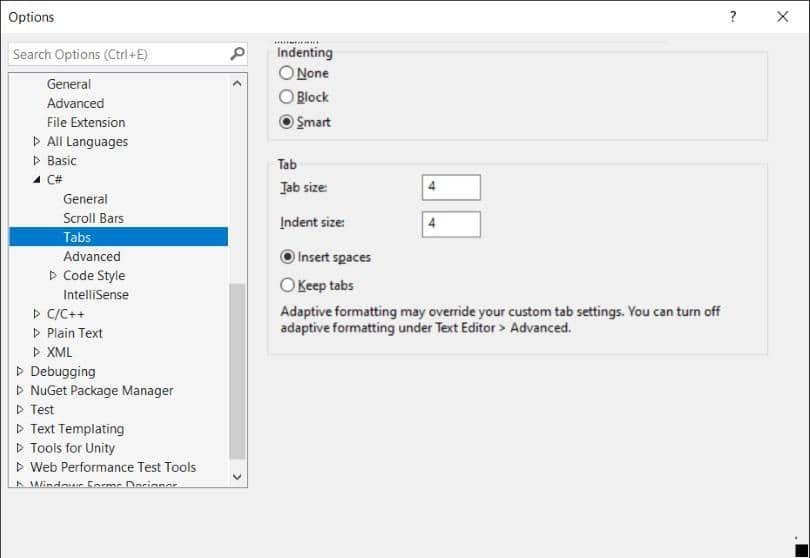
均一なインデントを決定する
インデントは通常 2 個または 4 個のスペースです。タブとスペースの論争を起こさずに、エディターの設定についてチーム全員の同意を得ましょう。
Visual Studio forWindowsで、[ツール] > [オプション] > [テキスト エディター] > [C#] > [タブ]に移動します。
Visual Studio forMacで、[環境設定] > [ソース コード] > [C# ソース コード]に移動します。テキスト スタイル を選択して設定を調整します。
注:Visual Studio には、タブをスペースに変換するオプションが用意されています。
括弧を省略しないでください
1 行のステートメントの場合でも、中括弧を省略しないでください。中括弧を使用すると一貫性が増し、コードの読みやすさと保守性が向上します。この例では、中括弧によってアクション DoSomething がループから明確に分離されています。
後でデバッグ行を追加したり、DoSomethingElse を 実行したりする必要がある場合は、中括弧がすでに配置されています。句を別の行に保持すると、ブレークポイントを簡単に追加できるようになります。
複数行のステートメントではわかりやすくするために中括弧を使用してください
ネストされた複数行のステートメントから中括弧を削除しないでください。この場合、中括弧を削除してもエラーは発生しませんが、混乱が生じる可能性があります。オプションであっても、わかりやすくするために中括弧を付けます。
スイッチ文を標準化する
フォーマットはさまざまであるため、スタイル ガイドにチームの設定を文書化し、それに応じて switch ステートメントを標準化します。
以下は、case ステートメントをインデントする例です。
水平間隔
スペースを入れるといった単純なことでも、画面上のコードの見栄えを良くすることができます。個人の書式設定の好みはさまざまですが、読みやすさを向上させるために以下の提案を考慮してください。
スペースを追加
コード密度を下げるにはスペースを追加します。余分な空白により、行の各部分が視覚的に分離されているという感覚が生まれます。
カンマの後のスペース
関数の引数間のコンマの後に 1 つのスペースを使用します。
括弧の後にスペースを入れない
括弧と関数の引数の後にスペースを追加しないでください。
関数と括弧の間にスペースを入れない
関数名と括弧の間にスペースを使用しないでください。
括弧内のスペースは避けてください
可能な限り、括弧内のスペースは避けてください。
フロー制御条件の前の間隔
フロー制御条件の前に 1 つのスペースを使用し、フロー比較演算子と括弧の間にスペースを追加します。
比較演算子によるスペース
比較演算子の前後に 1 つのスペースを使用します。
読みやすさのヒント
行を短くし、水平方向の空白を考慮してください。標準的な行幅 (80 ~ 120 文字) を決定し、長い行をオーバーフローさせずに小さな文に分割します。
前述したように、インデント/階層を維持するようにしてください。コードをインデントすると、読みやすさが向上します。
読みやすさのために必要な場合を除き、列の配置を使用しないでください。このタイプのスペースは変数を揃えますが、タイプと名前の組み合わせが複雑になる可能性があります。
ただし、列の配置は、ビット単位の式や大量のデータを持つ構造体では役立ちます。ただし、項目を追加すると、列の配置を維持するための作業が増える可能性があることに注意してください。一部の自動フォーマッタでは、列のどの部分が配置されるかが変更される場合があります。
垂直間隔と領域
垂直方向の間隔も活用できます。スクリプトの関連部分をまとめ、空白行を有効に活用します。コードを上から下に整理するには、次の操作を試してください。
- 依存するメソッドや類似するメソッドをグループ化します。コードは論理的で一貫性がある必要があります。同じことを実行するメソッドを隣り合わせに保つと、ロジックを読む人がファイル内を移動する必要がなくなります。
- 垂直の空白を使用して、クラスの異なる部分を区切ります。たとえば、次の間に 2 つの空白行を追加できます。
- 変数宣言とメソッド
- クラスとインターフェース
- if-then-else ブロック (読みやすさに役立つ場合)
これを最小限に抑え、可能であればスタイル ガイドで追跡してください。
コード内での領域の使用
#region ディレクティブを使用すると、C# ファイル内のコード セクションを折りたたんで非表示にすることができ、大きなファイルを管理しやすく、読みやすくすることができます。
ただし、このガイドのクラスに関する一般的なアドバイスに従えば、クラスのサイズは管理可能になり、#region ディレクティブは不要になります。コード ブロックを領域の背後に隠すのではなく、コードを小さなクラスに分割します。ソース ファイルが短い場合は、リージョンを追加する可能性が低くなります。
注:多くの開発者は、リージョンを コードの臭いやアンチパターンであると考えています。チームとして、議論のどちらの側に立つかを決めます。
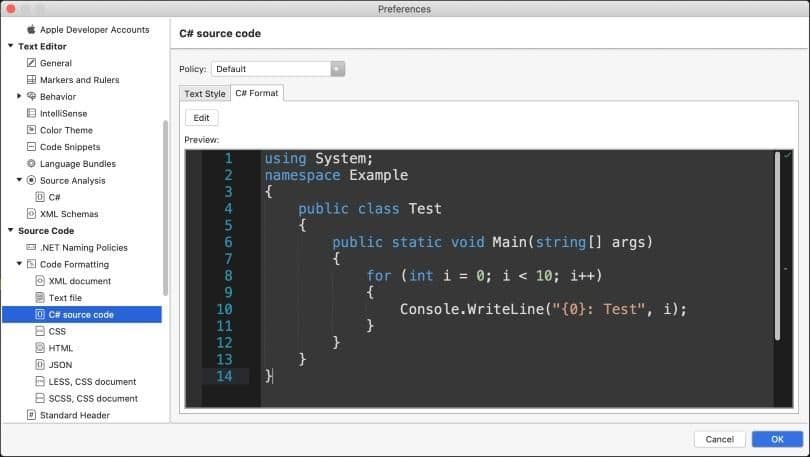
Visual Studio でのコードフォーマット
これらの書式設定ルールが難しそうに思えても絶望しないでください。最新の IDE を使用すると、設定と適用が効率的になります。書式設定ルールのテンプレートを作成し、プロジェクト ファイルを一度に変換できます。
スクリプト エディターの書式設定ルールを設定するには:
- Visual Studio forWindowsで、[ツール] > [オプション]に移動し、[テキスト エディター] > [C#] > [コード スタイルの書式設定]を見つけます。
- 設定を使用して 、全般、インデント、改行、間隔、折り返しの オプションを変更します。
- Visual Studio forMacで、[Visual Studio] > [基本設定]を選択し、[ソース コード] > [コードの書式設定] > [C# ソース コード]に移動します。
- 上部の ポリシー を選択し、「テキスト スタイル」 タブに移動します。C# の [フォーマット] タブで、 インデント、改行、間隔、折り返しの設定を調整します。
スクリプト ファイルを強制的にスタイル ガイドに準拠させるには:
- Visual Studio forWindowsで、[編集] > [詳細設定] > [ドキュメントのフォーマット] (Ctrl + K、Ctrl + D ホットキー) に移動します。空白とタブの配置のみをフォーマットする場合は、エディターの下部にある 「コード クリーンアップの実行」(Ctrl + K、Ctrl + E) を使用することもできます。
- Visual Studio for Macで、[編集] > [ドキュメントのフォーマット] (Ctrl + I ホットキー) に移動します。
Windows では、[ツール] > [設定のインポートとエクスポート]からエディター設定を共有することもできます。スタイル ガイドの C# コード形式でファイルをエクスポートし、チーム メンバー全員にそのファイルをインポートしてもらいます。
Visual Studio は、スタイル ガイドに準拠するのに役立ちます。すると、ホットキーを使用するだけで簡単にフォーマットできるようになります。
注:Visual Studio 設定をインポートおよびエクスポートする代わりに、EditorConfig ファイル (上記参照) を構成できます。これを行うと、異なる IDE 間でフォーマットを共有しやすくなります。また、バージョン管理を操作できるという利点もあります。詳細については 、.NET コード スタイル ルール オプションを 参照してください。
これはクリーン コードに限ったことではありませんが、Visual Studio を使用して Unity でのプログラミング ワークフローを高速化する 10 の方法を確認してください。これらの生産性に関するヒントを適用すると、クリーンなコードをフォーマットしてリファクタリングする方が便利になることを覚えておいてください。
コードスタイルのヒントをもっと知る
命名規則の詳細については 、ここを 参照するか、 電子書籍の完全版をご覧ください。詳細な コード スタイル ガイドの例も参照してください。